URL : URL parser
The url
module is used to process and parse URL.
A URL string is a structured string containing multiple meaningful components. When parsed, a URL object is returned containing properties for each of these components.
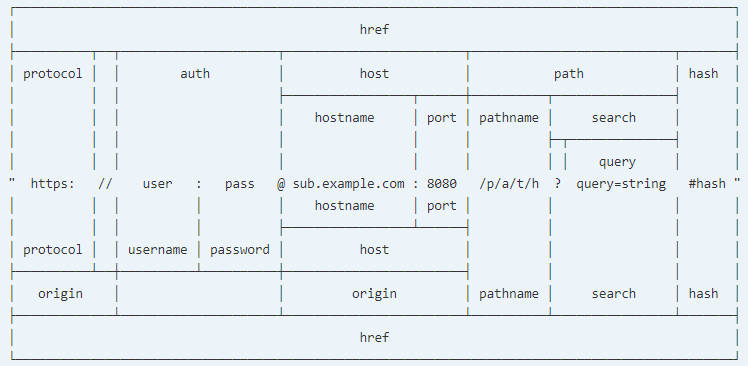
User can use the following code to import the url
module.
var URL = require('url');
Support
The following shows url
module APIs available for each permissions.
User Mode | Privilege Mode | |
---|---|---|
URL | ● | ● |
URL.parse | ● | ● |
URL.format | ● | ● |
URL.pathToFileURL | ● | ● |
URL.fileURLToPath | ● | ● |
url.hash | ● | ● |
url.host | ● | ● |
url.hostname | ● | ● |
url.port | ● | ● |
url.pathname | ● | ● |
url.search | ● | ● |
url.origin | ● | ● |
url.password | ● | ● |
url.username | ● | ● |
url.protocol | ● | ● |
url.href | ● | ● |
url.toString | ● | ● |
url.toJSON | ● | ● |
URL Class
new URL(input[, base])
input
{String} The absolute or relative input URL to parse. Ifinput
is relative, thenbase
is required. Ifinput
is absolute, thebase
is ignored.base
{String} | {URL} Thebase
URL to resolve against if theinput
is not absolute.- Returns: {URL} URL object.
Creates a new URL object by parsing the input
relative to the base
. If base
is passed as a string, it will be parsed equivalent to new URL(base)
.
Example
const myURL = new URL('https://example.org/foo');
const myURL = new URL('/foo', 'https://example.org/');
// https://example.org/foo
URL.parse(urlString[, parseQueryString[, slashesDenoteHost]])
urlString
{String} The URL string to parse.parseQueryString
{Boolean} Iftrue
, the query property will always be set to an object returned by thequerystring
module'sparse()
method. Iffalse
, the query property on the returned URL object will be an unparsed, undecoded string. default: false.slashesDenoteHost
{Boolean} Iftrue
, the first token after the literal string//
and preceding the next/
will be interpreted as thehost
. For instance, given//foo/bar
, the result would be{host: 'foo', pathname: '/bar'}
rather than{pathname: '//foo/bar'}
. default: false. EdgerOS 1.5.5 and later versions support.- Returns: {Object} URL object.
The URL.parse()
method takes a URL string, parses it, and returns a URL object. The URL object contains the following members:
protocol
{String} URL protocol scheme.auth
{String} User authentication information.'user:pass'
host
{String} Host portion of the URL.hostname
{String} Host name portion of the URL. The key difference betweenurl.host
andurl.hostname
is thaturl.hostname
does not include the port.path
{String} Path portion of the URL.pathname
{String} Path portion of the URL. The key difference betweenurl.path
andurl.pathname
is thaturl.pathname
does not include the query string.query
{String} | {Object} Query field. IfparseQueryString
istrue
, the query property will always be set to an object returned by thequerystring
module'sparse()
method. Iffalse
, the query property on the returned URL object will be an unparsed, undecoded string.search
{String} Raw query string include'?'
.fragment
{String} Fragment portion of the URL.hash
{String} Raw hash string include'#'
.slashes
{Boolean} With a value oftrue
if two ASCII forward-slash characters (/
) are required following the colon in the protocol. EdgerOS 1.5.5 and later versions support.
Example
console.inspectEnable = true;
console.log(URL.parse('https://user:pass@sub.host.com:8080/p/a/t/h?query=string#hash'));
URL.format(url[, options])
url
{Object} URL object create byURL.parse()
.options
{Object} Format options. Default value will be used whenoptions
isnull
orundefined
.auth
{Boolean}true
if the serialized URL string should include the username and password,false
otherwise. default: true.fragment
{Boolean}true
if the serialized URL string should include the fragment,false
otherwise. default: true.search
{Boolean}true
if the serialized URL string should include the search query,false
otherwise. default: true.
- Returns: {String} URL string.
Create a customizable serialization of a URL String representation of a URL object.
Example
console.log(URL.format({
protocol: 'https',
hostname: 'example.com',
pathname: '/some/path',
query: {
page: 1,
format: 'json'
}
}));
// https://example.com/some/path?page=1&format=json
URL.pathToFileURL(path)
path
{String} File path.- Returns: {Object} URL object.
Create a local file URL object.
Example
console.log(URL.format(URL.pathToFileURL('./file.txt'));
// file://./file.txt
URL.fileURLToPath(url)
url
{String} | {Object} URL, protocol must be'file'
.- Returns: {String} File path.
Get file path by specified URL.
Example
console.log(URL.fileURLToPath('file://./file.txt'));
// ./file.txt
URL Object
This object is create by new URL()
. This object is a smart object, modify a member of the object, other related members will automatically adjust the consistency according to the modified content.
url.hash
- {String}
Gets and sets the fragment portion of the URL.
Example
const myURL = new URL('https://example.org/foo#bar');
console.log(myURL.hash);
// Prints #bar
myURL.hash = 'baz';
console.log(myURL.href);
// Prints https://example.org/foo#baz
url.host
- {String}
Gets and sets the host portion of the URL.
Example
const myURL = new URL('https://example.org:81/foo');
console.log(myURL.host);
// Prints example.org:81
myURL.host = 'example.com:82';
console.log(myURL.href);
// Prints https://example.com:82/foo
Invalid host values assigned to the host
property are ignored.
url.hostname
- {String}
Gets and sets the host name portion of the URL. The key difference between url.host
and url.hostname
is that url.hostname
does not include the port.
Example
const myURL = new URL('https://example.org:81/foo');
console.log(myURL.hostname);
// Prints example.org
myURL.hostname = 'example.com:82';
console.log(myURL.href);
// Prints https://example.com:81/foo
Invalid host name values assigned to the hostname
property are ignored.
url.port
- {String}
Gets and sets the port portion of the URL.
The port value may be a number or a string containing a number in the range 0
to 65535
(inclusive). Setting the value to the default port of the URL
objects given protocol
will result in the port
value becoming the empty string (''
).
The port value can be an empty string in which case the port depends on the protocol/scheme:
protocol | port |
---|---|
"ftp" | 21 |
"file" | |
"gopher" | 70 |
"http" | 80 |
"https" | 443 |
"ws" | 80 |
"wss" | 443 |
Example
const myURL = new URL('https://example.org:8888');
console.log(myURL.port);
// Prints 8888
// Default ports are automatically transformed to the empty string
// (HTTPS protocol's default port is 443)
myURL.port = '443';
console.log(myURL.port);
// Prints the empty string
console.log(myURL.href);
// Prints https://example.org/
myURL.port = 1234;
console.log(myURL.port);
// Prints 1234
console.log(myURL.href);
// Prints https://example.org:1234/
// Completely invalid port strings are ignored
myURL.port = 'abcd';
console.log(myURL.port);
// Prints 1234
// Out-of-range numbers which are not represented in scientific notation
// will be ignored.
myURL.port = 1e10; // 10000000000, will be range-checked as described below
console.log(myURL.port);
// Prints 1234
url.pathname
- {String}
Gets and sets the path portion of the URL.
Example
const myURL = new URL('https://example.org/abc/xyz?123');
console.log(myURL.pathname);
// Prints /abc/xyz
myURL.pathname = '/abcdef';
console.log(myURL.href);
// Prints https://example.org/abcdef?123
url.search
- {String}
Gets and sets the serialized query portion of the URL.
Example
const myURL = new URL('https://example.org/abc?123');
console.log(myURL.search);
// Prints ?123
myURL.search = 'abc=xyz';
console.log(myURL.href);
// Prints https://example.org/abc?abc=xyz
url.origin
- {String}
Gets the read-only serialization of the URL's origin.
Example
const myURL = new URL('https://example.org/foo/bar?baz');
console.log(myURL.origin);
// Prints https://example.org
url.password
- {String}
Gets and sets the password portion of the URL.
Example
const myURL = new URL('https://abc:xyz@example.com');
console.log(myURL.password);
// Prints xyz
myURL.password = '123';
console.log(myURL.href);
// Prints https://abc:123@example.com
url.username
- {String}
Gets and sets the username portion of the URL.
Example
const myURL = new URL('https://abc:xyz@example.com');
console.log(myURL.username);
// Prints abc
myURL.username = '123';
console.log(myURL.href);
// Prints https://123:xyz@example.com/
url.protocol
- {String}
Gets and sets the protocol portion of the URL.
Example
const myURL = new URL('https://example.org');
console.log(myURL.protocol);
// Prints https:
myURL.protocol = 'ftp';
console.log(myURL.href);
// Prints ftp://example.org/
url.href
- {String}
Gets and sets the serialized URL.
Example
const myURL = new URL('https://example.org/foo');
console.log(myURL.href);
// Prints https://example.org/foo
myURL.href = 'https://example.com/bar';
console.log(myURL.href);
// Prints https://example.com/bar
url.toString()
- Returns: {String} URL string.
The toString()
method on the URL
object returns the serialized URL. The value returned is equivalent to that of url.href
and url.toJSON()
.
url.toJSON()
- Returns: {String} URL string.
The toJSON()
method on the URL
object returns the serialized URL. The value returned is equivalent to that of url.href
and url.toString()
.
Example
const myURLs = [
new URL('https://www.example.com'),
new URL('https://test.example.org')
];
console.log(JSON.stringify(myURLs));
// Prints ["https://www.example.com/","https://test.example.org/"]